Patrick Kennedy
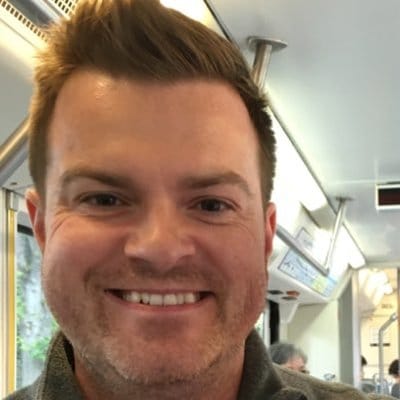
Patrick is a software engineer from the San Francisco Bay Area with experience in C++, Python, and JavaScript. His favorite areas of teaching are Vue and Flask. In his free time, he enjoys spending time with his family and cooking.
Developing Web Applications with Python and Flask!
This course teaches the fundamentals of Flask by building a full-featured web application for managing a stock portfolio. Each chapter builds upon itself to achieve the goal of developing a web app.
The first part focuses on those core pieces:
Additionally, you'll learn how to write tests for a Flask application using pytest.
request
proxyThe second part focuses on structuring a Flask application using blueprints.
In this part, you'll learn how to create different configurations for different environments -- e.g., development, testing, and production. We'll dive into how the Application Factory design pattern can be applied to Flask to easily create a Flask application as well.
This part also provides a deep-dive into how Flask processes requests and how the application and request contexts are handled during a request.
Finally, you'll learn how fixtures in pytest can help create the initial state for running tests.
The third part focuses on using Flask-SQLAlchemy to:
In addition, we'll look at how to create custom CLI commands in Flask to populate the database with data.
Starting with the fourth part, we'll utilize Test-driven Development (TDD) to incrementally add functionality to the Flask app.
This part focuses on managing the users of the app:
In addition, you'll learn about how to mitigate CSRF and XSS attacks.
The fifth part focuses on working with stock data.
We'll look at how to display the stock portfolio for each user and how to add new stocks.
You'll learn about monkeypatching for testing an external API. Then, we'll dive into pulling in stock data from an external API.
This part culminates with creating charts of the historical stock prices using Chart.js.
The sixth part focuses on deploying the app to Render.
This course is intended for people with some experience with Python. No prior experience with other web frameworks (Django, Pyramid, FastAPI, etc.) is required.
I'm enjoying Patrick Kennedy's Developing Web Applications with Python and Flask course. I love the in-depth explanations of how Flask works under the hood, and I'm switching from unittest to Pytest as well. TestDriven.io is definitely the best site for teaching unit/integration/functional testing.
I would like to thank you very much for the Developing Web Applications with Python and Flask course. Each concept was nicely explained and everything was clear. I have gone through several Flask courses and this course was the best.
The Developing Web Applications with Python and Flask course was absolutely amazing, I learned best practices for Flask development and Test-Driven Development in general. I work as an ML engineer and decided to take this course as I wanted to learn more about web development. Although the work that I do during the day is much different from web development, I learned a number of things that I can use in that context. I recommend this course to not only those wanting to learn Flask, but for those who want to incorporate Test-Driven Development into their everyday development workflow in general.
The TestDriven.io courses are some of the best courses I've ever done for any language, any platform, any price range... just some of the most thorough and well-sourced courses around.
I am very much into buying and purchasing any course by you and your team. I've never felt like a better programmer ready to show my coding chops to the world.
What tools and technologies are used in this course?
This course covers a variety of technologies and services:
What will you build?
In this course, you'll build an app for tracking stock portfolios. You can think of it as a basic version of a brokerage site like Fidelity or TD Ameritrade. The app provides user management functionality (e.g., registration, login/logout, password reset) so that multiple users can access the app. After registering, users will be able to view and add new stocks to their portfolio.
An example of the Flask application that you'll be creating in this course can be found at:
Demo:
What support does TestDriven.io offer?
Since the courses mimic real-world development, support is provided via Stack Overflow. Helpful users, including the developers of the courses, read and respond to messages on Stack Overflow. If you get stuck and you can't find an answer via Stack Overflow, feel free to reach out via email directly. Just be sure to detail what you've tried. For more, review Support and Consulting.
How long does it take to complete the course?
It's dependent on your current skill level. On average, it takes approximately 12 hours to complete.