Developing a Real-Time Taxi App with Django Channels and React
- Updated November 10th, 2022
- v4.0.0
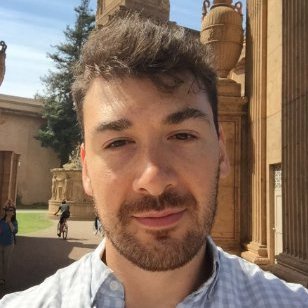
Learn how to develop and test a real-time ride-sharing app with Django Channels, React, and Cypress!
In this course, you'll learn how to create a ride-sharing application that incorporates a React frontend with a Django backend in a Docker container. The focus of this course is the real-time communication between client and server. We'll be using Django Channels and Redis to send and receive JSON messages over an open WebSockets connection.
Another important aspect of these chapters is Test-Driven Development (TDD). Each step of the way, we'll demonstrate how to test both the UI and the APIs.
What will you learn?
Select a Part
In Part 1, you'll learn how to program the server code of the ride-sharing application. We'll start by developing a custom user authentication model and data profile. Then we'll create a data model to track the trips that the riders and drivers participate in along with the APIs that provide access to that data. Lastly, we'll leverage the asynchronous nature of Django Channels to send and receive messages via WebSockets. Throughout this part, we'll be testing every function to make sure that the code we write operates the way we expect it to.
Learning Objectives
- Create a RESTful API with Django REST Framework
- Implement token-based authentication with JSON Web Tokens (JWT)
- Use Django Channels to create and update data on the server
- Send messages to the UI from the server via WebSockets
- Test asyncio coroutines with pytest
In Part 2, you'll take the first steps in setting up the user interface for the app. We'll start by creating a React frontend application. Using JSX, we'll write components and services to complement the authentication APIs that allow users to sign up, log in, and log out. Again, we'll make sure to test our application along the way, this time using the Cypress end-to-end testing framework. Before we end this part, you'll learn how to run both the frontend and backend in a single Docker container.
Learning Objectives
- Create a React app from scratch using Create React App
- Make functional React components that use React Hooks
- Configure routing to navigate between different views in your app
- Write unit and end-to-end tests with Cypress to confirm your code is working as expected
- Mock AJAX requests with Cypress
- Implement Bootstrap to polish the look-and-feel of your UI
- Code forms that send data to the server asynchronously
- Run the server and the client as Docker services
- Employ authentication so end users can sign up, log in, and log out of the application
In Part 3, you'll finish coding the frontend and stitch the UI together with the server APIs. Continuing where we left off in Part 2, we'll expand our UI to build two dashboards -- one for the rider and one for the driver. Here, we'll also create the JSX code necessary for establishing a WebSockets connection with the server and subscribing to it. Along with manual testing, we'll test the real-time nature of the app through automated tests. We'll also incorporate Google Maps so that users can visualize their current locations and the addresses they input.
Learning Objectives
- Perform side effects in React functional components with the Effect Hook (
useEffect
) - Use WebSockets in React
- Add toast notification messages to React
- Integrate the Google Maps API into a React application
What do you need to know?
This is not a beginner course. It's designed for the advanced-beginner -- someone with at least six months of web development experience. Before beginning, you should have some familiarity with the following topics. Refer to these resources for more info:
Django
- Test-Driven Development with Python, Django, and Docker
- Looking for a solid introduction to Django? Check out Django for Beginners. Highly recommended!
React
What developers are saying
What I really enjoyed about the Developing a Real-Time Taxi App with Django Channels and React course was learning how to develop a cohesive, multi-tier application. I've spent time learning React and Django here and there, but this course put everything together -- not only with a finished app but it also helped me apply test-first design with Cypress. I also learned how powerful Docker is, and how easy it is to get multi-tier architecture up and running on a single machine and make code changes that sync with the code in the container that can be tested in real-time. All this while learning how to build an API with Django REST Framework and add streaming capabilities with Django Channels. And then getting React up and running and organized in a structured format and having to overcome some harder problems like authentication, authorization, and CORS. An amazing course. I highly recommend it!
The TestDriven.io courses are some of the best courses I've ever done for any language, any platform, any price range... just some of the most thorough and well-sourced courses around.
Just a word of thanks for doing such a great job with these training courses. The thorough, entire-lifecycle approach -- from implementation through test, coverage, quality, CI/CD, and all the rest -- is what separates these courses from other training material that I've completed. I'll be able to walk away from here with knowledge and skills that I can apply immediately at work -- and for that I'm grateful. It's a rare gift in an environment where so much 'training' is really just lightweight treatment that doesn't begin to scratch the surface of real, end-to-end software development. Really well done!
The TestDriven.io courses are worth 10 times what I paid for them.
I am very much into buying and purchasing any course by you and your team. I've never felt like a better programmer ready to show my coding chops to the world.
Frequently Asked Questions
What will you build?
Django app: We'll configure user authentication and authorization with core Django, coordinate two-way messaging with Django Channels and Redis, and construct a RESTful API with Django REST Framework.
React app: We'll build an number of React components, using React Hooks, and control the flow with routing; communicate with the backend using WebSockets, React services, and reactive programming; and leverage third-party apps like Google Maps to bolster the user experience.
Demo:
What tools and technologies are used in this course?
This course covers a variety of technologies and services:
Back-end
- Python
- Django
- Django REST Framework
- Django Channels
- Postgres
- Redis
- JSON Web Tokens (JWTs)
- pytest
Front-end
- JavaScript
- React
- React Hooks
- Formik
- Cypress
- WebSockets
- Geolocation
Tools
- Docker
- Google Maps
What support does TestDriven.io offer?
Since the courses mimic real-world development, support is provided via Stack Overflow. Helpful users, including the developers of the courses, read and respond to messages on Stack Overflow. If you get stuck and you can't find an answer via Stack Overflow, feel free to reach out via email directly. Just be sure to detail what you've tried. For more, review Support and Consulting.
How long does it take to complete the course?
It's dependent on your current skill level. On average, it takes approximately 12 hours to complete.